C Programming
History of C Language
Since 1950, FORTRAN is using for scientific application and COBOL for Business applications. A research for general purpose language has started.
CPL – Common Programming Language
BCPL – Basic Common Programming Languages
B – First Letter in BCPL
C – Second Letter in BCPL.
C was developed in AT&T’s Bell Laboratory in 1972 by Dennis Ritchie. C was originally developed for use in UNIX platform. Later, entire UNIX operating system was re-written by C Language.
C is general purpose, structured programming Language.
Features of C
- C can be used to develop system as well as application software.
- Portable
- Supports variety of data types like int, float etc
- Procedure Oriented Programming Language
- Provides built-in functions
- Execution is fast than other high level language programs.
- Middle level Language
History of C Language
Since 1950, FORTRAN is using for scientific application and COBOL for Business applications. A research for general purpose language has started.
CPL – Common Programming Language
BCPL – Basic Common Programming Languages
B – First Letter in BCPL
C – Second Letter in BCPL.
C was developed in AT&T’s Bell Laboratory in 1972 by Dennis Ritchie. C was originally developed for use in UNIX platform. Later, entire UNIX operating system was re-written by C Language.
C is general purpose, structured programming Language.
Features of C
- C can be used to develop system as well as application software.
- Portable
- Supports variety of data types like int, float etc
- Procedure Oriented Programming Language
- Provides built-in functions
- Execution is fast than other high level language programs.
- Middle level Language
Basic Structure of the C Program
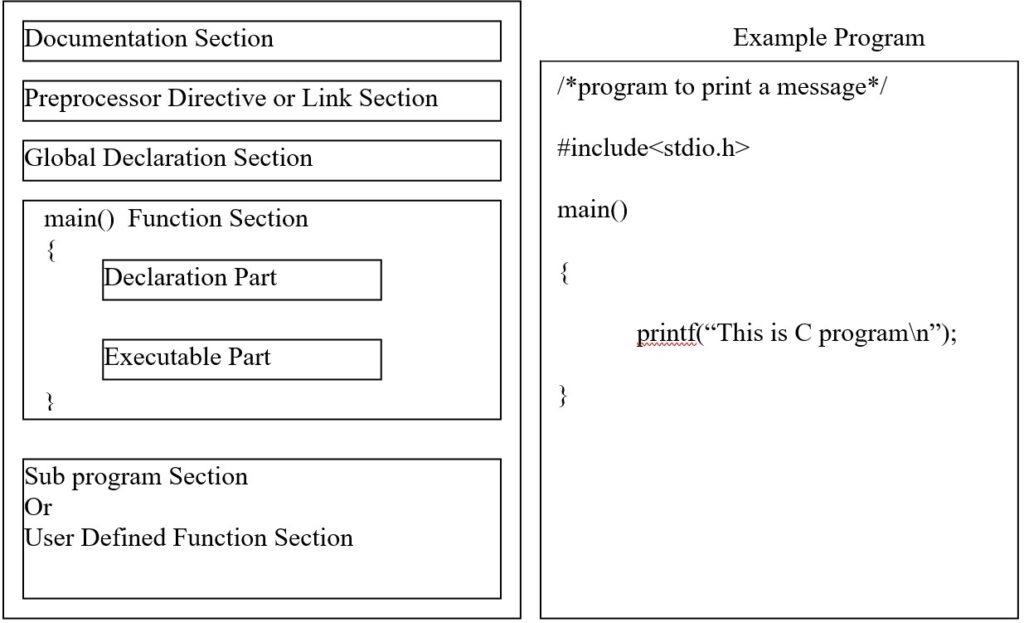
#include line in the above program is a reference to a special file called stdio.h (called the header file contains predefined programs for standard input and output functions). Any statement starting with # is called preprocessor directive.
main() is the starting function which contains executable statements in between two curly braces { and }. Curly brackets are used to group statements together as a function or in the body of loop. Such group is called as compound statement or block.
Important points to remember:
- Every C program requires a main() function. Use of more than one main() is illegal. The place of main() is where the program execution begins.
- The Execution of the function begins at the opening brace and ends at the closing brace.
- C programs are written in lowercase letters. However uppercase letters may be used for symbolic names and constants.
- All the words in a program line must be separated from each other by at least one space or a tab, or a punctuation mark.
- Every statement must end with a semicolon.
- All variables must be declared for their type before they are used in the program.
- Compiler directives such as define and include are special instructions to the compiler, so they do not end with a semicolon.
- When braces are used in the program make sure that the opening brace has corresponding ending brace.
- C is a free form language and therefore proper form of indentation of various sections would improve the legibility of the program.
A comment can be inserted almost anywhere a space can appear. Use of appropriate comments in proper places increases the readability of the program and helps in debugging and testing.
TYPES OF ERRORS
Saving C Program:
C program can be edited, compiled and run in many platforms like DOS, UNIX or directly in the editor provided by their flavor of compiler (like Turbo C). C program code (Source Code) to be saved with file extension .c
COMPILING C PROGRAM:
Compiling means converting Source code (program code written in high level language) into object code (code format understandable by Computer). Compilation process checks the source code for syntax or semantic errors, reports about errors and finally creates object code file with extension .obj only when no syntax or semantic errors.
Syntax errors are the errors occurred when a statement in the program had not been written by following syntax rules of C language.
Example :
/*program to print a message*/
#include<stdio.h
main()
{
Printf(“This is C program\n”)
When compiled the following errors occur:
Error test.c 1: No file name ending
Error test.c 5: Statement missing ;
Error test.c 6: compound statement missing }
Semantic errors are displayed as warnings and do not break compilation process.
Example: if you have declared a variable and not used the same in the program, compiler gives a warning that “Code has no effect”
LINK AND RUN C PROGRAM:
Linking is the process of getting included header files to tie with current program. The output of Linking is the executable program file with extension .exe
LINKER ERRORS
Executable file can only be created when there are no linker errors. Linker errors occur when certain header file is not found.
LOGICAL AND RUNTIME ERRORS:
Logical Errors cannot be found out by Compiler or Linker. This kind of errors are called logical errors can only removed by debugging. Debugging is the process of checking program step by step and verifies the results.
Example:
/*Program to compute average of three numbers*/
#include
main()
{
int a=10,b=5,c=20,sum,avg;
sum=a+b+c;
avg=sum/3;
printf(“The average is %d\n”,avg);
}
This program gives output as 8 but actual average is 8.33 because of rounded off error. To rectify the error we have declare avg as float data type.
Another kind of Errors called Run time Errors like program do not producing the result, execution stops in between and error message flashes on the screen.
Example:
/*program to divide a sum of two numbers by their difference*/
#include
main()
{
int a=10,b=10;
float c;
c=(a+b)/(a-b);
printf(“The value of the result is %f\n”,c);
}
This program gives run time error as Divide by Zero
LANGUAGE FUNDAMENTALS
Character Set: The Character set of C Language has alphabets, numbers and special symbols as shown below:
- Alphabets including lower and upper case letters
- Numbers 0-9
- Special Characters like ; , : { / \ ^ # @
Keywords are reserved words which have standard, predefined meaning. They can also be called as program-defined Identifier. They should be use in lower case. They are 32 in number.
List of C keywords:
char while do typedef auto
int if else switch case
printf double struct break static
long enum register extern return
union const float short unsigned
continue for signed void default
goto sizeof volatile
Data type is the format of data elements that are stored in the program. A datatype determines:
- Type of data
- permissible range of values that it can store
- Memory requirement
Four Basic data types in C Language: int, char, float and Double
Program Element is a basic building block that is used to construct program. Program Elements are two types:
- Data element Eg: variable, array
- Code element Eg: function
Identifier is the name given to various program elements such as constants, variables, functions and arrays etc.
Rules for naming an Identifier:
- It consists of letters and numbers
- First character must be an alphabet or underscore
- Both upper and lower case are allowed. Eg: TEXT is different from text
- No special symbols are allowed
Examples of Valid Identifiers: X, X123, _XI, temp, tax_rate
Examples of Invalid Identifiers: 123, “X”, Order-no, error flag
VARIABLES AND CONSTANTS
Data Element is a type of program element that can enable us to store data in memory.
In this lesson we are discussing about two such data elements that are variable and constant.
Variable is a memory location referring with an identifier whose value changes from time to time during program execution.
Declaration of Variables: Declaration associates a group of identifiers with a specific data type. We can understand declaration as an introduction of an identifier with its characteristics.
Example of Variable Declaration statements:
int a;
float r1,r2;
CONSTANT:
A constant is a memory location referred by an identifier whose value cannot be changed throughout the execution of a program.
Declaration of constant can be done with the following syntax:
const =’value’;
Again, the values that can be stored inside the memory location referring by a variable or constant also known as Constants.
Based on the four data types we have four types of constant values:
- Integer Constants Eg: 32727, 45, 8
- Floating Point Constants Eg: 2.58, 56.0
- Character Constants Eg: ‘A’, ‘c’, ‘u’
- String Constants Eg: “Rama”, “blue sea”, “ABC123”
Rules to form Integer and Floating Point Constants
- No comma or blank space is allowed in a constant.
- It can be preceded by – (minus) sign if desired.
- The value should lie within a minimum and maximum permissible range decided by the word size of the computer.
Symbolic Constant is a name associated with a sequence of characters or any basic type of constant. Symbolic constant is replaced with the constant at the time of compilation.
Symbolic Constants can be defined with the following preprocessor statement:
#define
Eg:
#define print printf
#define MAX 100
#define TRUE 1
#define FALSE 0
#define SIZE 10
INITIALISATION
Storing data into a variable can be done in two ways:
- Initialization
- Along with declaration
- Assignment statement
- User input
But, storing data into a constant can be done in two ways:
- Initialization along with declaration
- User Input
Using Assignment operation to initialise a constant leads to compile time error.
Initialisation is the process of storing some value in the data element by the developer. If the data element is left with out storing any value by using any one of the above methods then the system will store some unpredictable value in the referred memory location.
//code showing how uninitialised variable show unpredicatble results
#include
void main()
{
int a,b;
printf(“Value of a is %d”,a);
printf(“Value of b is %d”,b);
printf(“Sum of the numbers is %d”,a+b);
}
The above code could successfully compile but would result some unexpected result. The result depends upon the system configuration, IDE and many other factors.
So, it is compulsory to have some value in the variable which was declared. As we mentioned earlier, initialization is the process of storing value by the developer. This can be done in two ways:
- Along with declaration
- Through assignment statement
A variable can be initialized to a value at the time of its declaration. The following is an example line showing how a variable can be initialized along with declaration:
int a=10;
A variable can also be initialized to a value later to its declaration with the help of an assignment statement.
Example of initialization with assignment:
int a;
a=10;
At this moment, it would be appropriate to discuss about assignment operation here. The general equal to operator ‘=’ is not equal to operator in C Language. It is known as assignment operator. Any program line or statement using ‘=’ operator is known as assignment statement.
Syntax of assignment statement:
Variable = value/variable/expression
As you can see in the above syntax, at the left hand side there must be a variable nothing else. The variable at left hand side must have been declared prior to this statement but may or may not have initalised. But on the other hand, at right hand side of the assignment statement we can see either a value or a variable which is already have value or an expression.
The following statements are the examples of 3 types of assignment statements we can see in C program:
a=10; //variable = value
b=a; //variable=variable already had value
c=a+b; //variable =expression. The result of the expression will be stored in the variable
DATA TYPES AND MODIFIERS
Four keywords out of 32 pre-defined keywords of C Language are used to specify the type of data of a data element or data acceptable or return by a function element. The four keywords are int, char, float and double.
The data type will be used at the time of declaration of a data element like variable, constant, array etc. Moreover, the data type is also used at the header of a function definition to specify the type of data a function can intake or the type of data that a function can return.
The following are the examples of usage of data types:
int a;
float a,b;
int add(int x, int y){….}
Basically a datatype specifies three things:
- Memory requirement
- Range of values
- Type of operations
DATA TYPE TYPE OF DATA MEMORY RANGE
Int Integer 2 Bytes − 32,768 to 32,767
Char character 1 Byte − 128 to 128
Float Floating point number 4 bytes 3.4e − 38 to 3.4e+38
Double Floating point number
with higher precision 8 bytes 1.7e − 308 to 1
Modifiers/Qualifiers for Data type:
Data type Size (bytes) Range
Short int/ int/signed int 2 −32768 to 32,767
Long int 4 −2147483648 to 2147483647
Unsigned int 2 0 to 65535
Signed char 1 −128 to 127
Unsigned char 1 0 to 255
Basic I/o Operations
Input is the process of getting data from the user and Output is the activity of displaying a message or a result of program to the user. Basically I/O operations make possible the communication between the user and the program. Input and Output operations performing with standard I/O devices that is keyboard and monitor are called as Standard I/O operations. There are some other type of I/O operations like file I/O operations which will be discussed later in this course.
In C Language, all the I/O is based on the functions only. Moreover those functions are pre-defined functions existed in the library of header files like stdio.h, conio.h etc. We have to attach the code of the respective functions to our program in order to use a i/o function. For example, to use printf and scanf functions we have to link their definitions from stdio.h to our program.
There are Two types of I/O Functions
- Formatted
- Unformatted
Formatted I/o Operations
The formatted I/O in C language can be done using two popular functions known as printf() and scanf().
Printf function
printf() stands for print formatted which has the following syntax:
Syntax1: printf(“string”);
Using the above syntax, we can print any text or phrase that is enclosed in between the two double quotes on the console to the user.
Syntax2: printf(“string [%formatspecifier]”,[variable]);
If you have used any format specifiers(like %d, %c etc) inside the double quotes of 1st argument of printf() function, then the place of the format specifier would be replaced with the corresponding variable value provided as 2nd argument while printing.
In other words, we can say there is no need of using format specifiers inside the text string of printf() function. In this case printf() function would have only one argument. But if we have used any format specifiers then the number of arguments that printf() function would have is depends upon the number of format specifiers inside the 1st argument.
Example:
printf(“[%formatspecifier1][%formatspecifier2] ….[%formatspecifierN]”, var1,var2,…,varN);
the place format specifier1 would be replaced with the value of var1 and specifier2 would be replaced with var2.
Two consecutive printf statements cannot print your specified text in multiple lines. It can only be done with the help of newline character.
For example check the following code:
printf(“First Line”);
printf(“Second Line”);
printf(“Third Line”);
The output of the above code would be : First LineSecond LineThird Line
From the above output we can understand text divided into multiple printf statements could not mean to the system that it has to be displayed in multiple lines. Change the same code with newline character as below to get your desired output.
printf(“First Line\n”);
printf(“Second Line\n”);
printf(“Third Line”);
OR
printf(“First Line\nSecond Line\nThird Line”);
Format Specifier
Format specifier is a special character followed by a percentile(%) symbol that is generally found any formatted function like printf() and scanf(). They are called as format specifiers because they are specifying the format of the data that must be accepted from the user or the format of data that should be displayed to the user.
The following are various format specifiers of C Language:
%c | Character |
%d, %i | signed decimal integers |
%f | decimal floating point |
%s | string of characters |
Escape Characters or Escape Sequences
Escape Characters or Escape Sequences
Another special character here that are used with printf statement also known as Escape Characters. These characters are prefixed with a backward slash(\) denotes a special meaning for printf to print output.
\b | Backspace |
\n | Newline |
\t | horizontal tab |
\” | double quote |
\’ | single quote |
\\ | Backslash |
\v | vertical tab |
scanf() function
Let us now continue with the second formatted input function scanf(). This is used to get input from the user using standard Input i.e. keyboard and store in a memory location referring by an identifier.
Syntax of scanf() function:
scanf(“[%format specifier1][%format specifier2]….[%formatspecifierN]”, var1,var2,…,varN);
//code to demonstrate all basic data types of C Language
#include
void main()
{
int a;
float b;
double c;
char d;
printf(“Enter any Character:”);
scanf(“%c”,&d);
printf(“Enter any Integer Value:”);
scanf(“%d”,&a);
printf(“Enter any Float Value:”);
scanf(“%f”,&b);
printf(“Enter any Double Value:”);
scanf(“%lf”,&c);
printf(“\n\nYour data is:\n”);
printf(“Integer value in a is %d\n”,a);
printf(“Float value in b is %f\n”,b);
printf(“Double value in c is %lf\n”,c);
printf(“Character value in d is %c”,d);
}
The printf() following scanf() function need no newline character since user will press Enter after his input. But printf() following another printf() needs newline character if you want to print the output in next line.
//check the following code to understand the working of printf followed by scanf
void main()
{
printf(“Enter a number:”);
int a;
scanf(“%d”,&a);
printf(“value of a is %d”,a);
}
//printf following by printf
void main()
{
printf(“First Line”);
printf(“Second Line”);
printf(“\nThird Line”);
printf(“\nFourth Line\nFifth Line”);
printf(“\nSixth Line”);printf(“\nSeventh Line”);
}
Unformatted I/O
Unformatted I/O in C language is done by the functions like getch, getche, putch, purchar() etc. These functions will be discussed later in this course in Strings Handling.
OPERATORS AND EXPRESSIONS
What is a program statement?
A program statement is a line that ends with some termination point and instructs the processor to do something.
How many types of program statements?
- Declaration statements
- Definitions or blocks or compound statements
- Assignment statements
- Expressions and conditions
What is an expression?
An expression is a line that consists of arithmetic operators and operands and usually returns a value got as a result from the evaluation of expression.
Eg: 2+3, (a-b)*2
What are Operators and Operands?
Operator is basically a symbol indicating an operation. Each operator specifying an operation to be done on some number of operands. Operands are the values on which the operation has to be performed.
For example in the arithmetic expression a+b
The + symbol is known as operator
And a,b are known as operands.
How many types of operators?
Based on the number of operands, operators in C language are categorized as:
- unary operators: works with single operand Eg: a++
- Binary operators: works with two operands Eg: a+b
- Ternary operators: works with three operands. There is only one ternary operator that is ?: which is also known as shorthand notation for if…else statement. So, we will discuss about this operator in selection statements chapter.
Based on the type of operation they perform operators are divided into:
- arithmetic operators
- assignment operators
- relational operators
- increment & decrement operators
- bit wise operators
- logical operators
Arithmetic Operators
As the name saying they are used to perform arithmetic operations on two operands. Arithmetic operators can work on numeric data types like int, float and double. All the arithmetic operators are binary operators.
Operator Symbol | Operation |
+ | Addition |
– | Subtraction |
* | Multiplication |
/ | Division |
% | Modulus (Integer Operator) |
Order of Precedence for Arithmetic Operators:
- Brackets evaluates first. In case of nested brackets, inner one evaluates first then outer.
- Multiplication, division and modulus have next precedence. If all or some of them are there, then evaluation is left to right.
- Addition and subtraction have last precedence. If both exists, evaluation is left to right.
Assignment Operators
The ‘equal to’ sign (=) is known as assignment operator in C language. The program statement that has assignment operator is known as assignment statement.
We have already discussed about the syntax and examples of assignment statements in data types and variable declaration chapter of this course.
The assignment operator can be combined with arithmetic operators to create shorthand notations.
For example, a=a+2; can be written as a+=2;
In the above expression we want to add 2 to the existing value of ‘a’ and again want to store back into a. In this case shorthand notations will help.
Like that, all the remaining arithmetic operators can be combined with assignment operator.
Type Casting
The value for the expression is promoted or demoted depending on the type of the variable on left hand side of = in the assignment statement.
For example, consider the following assignment statements:
int i;
float b;
i = 4.6;
b = 20;
In the first assignment statement, float (4.6) is demoted to int. Hence i gets the value 4. In the second statement int (20) is promoted to float, b gets 20.0.
If we have a complex expression like:
float a, b, c;
int s;
s = a * b / 5.0 * c;
Where some operands are integers and some are float, then int will be promoted or demoted depending on left hand side operator. In this case, demotion will take place since s is an integer.
This is also known as implicit type conversion or type casting. There is a way to explicitly convert the data type of a value.
int x=7, y=5;
float z;
z=x/y; /*Here the value of z is 1*/z = (float)x/(float)y; /*Here the value of z is 1.4*/
Increment & Decrement Operators
Increment and Decrement Operators are the unary operators of C language. These can be used as a shorthand notation to add 1 or subtract 1 from the existing value of a variable.
The ++ is known as the Increment operator and — is known as the Decrement operator. The difference between increment/Decrement operators and shorthand notation of assignment operator is, we can use shorthand notation for any operator and for any value but we can use increment/decrement operators for addition and subtraction that too for addition or subtraction of 1 only.
For example,
a=a+1; can be written as a+=1 or a++
a=a+2; can be written as a+=2 but cannot use increment operator.
a=a*8 can be written as a*=8 but there is no other choice
The operand of Increment/Decrement operator must be variable but not a constant/expression.
What is Post/Pre Increment/Decrement?
If an increment / decrement operator is written before a variable, it is referred to as preincrement / predecrement operators and if it is written after a variable, it is referred to as post increment / postdecrement operator.
Expression Explanation
++a Increment a by 1, then use the new value of a
a++ Use value of a, then increment a by 1
–b Decrement b by 1, then use the new value of b
b– Use the current value of b, then decrement by 1
The precedence of these operators is right to left.
Example:
int a = 2, b=3,c;
c = ++a – b- -;
printf (“a=%d, b=%d,c=%d\n”,a,b,c);
OUTPUT
a = 3, b = 2, c = 0.
Example2:
int a = 1, b = 2, c = 3,k;
k = (a++)*(++b) + ++a – –c;
printf(“a=%d,b=%d, c=%d, k=%d”,a,b,c,k);
OUTPUT
a = 3, b = 3, c = 2, k = 6
Relational Operators
Relational Operators are used to compare values of two variables. These operators are used to form conditions that can yield out either true or false. Conditions are usually can be seen with selections, loops in C language.
Relational Operator | Condition | Meaning |
== | x==y | x is equal to y |
!= | x!=y | x is not equal to y |
< | x<y | x is less than y |
<= | x<=y | x is less than or equal to y |
> | x>y | x is greater than y |
>= | x>=y | x is greater or equal to y |
Logical Operators
Logical Operators are generally used to combine two or more conditions and evaluates to True or False. But Logical operators work with conditions that can return a boolean value (i.e. True or False) and even variables and constant values too. When used with other than conditions, Logical operators treat all non-zero values as True and ‘0’ as false.
Logical Operator | Meaning | Evaluation Procedure |
&& | AND | Evaluates to true only if all conditions are true |
|| | OR | Evaluates to true any one condition is true |
! | NOT | Evaluates true if condition is false and vice versa |
Logical AND
Logical AND (&&) checks two boolean values and returns either True or False. The following is the table showing how Logical AND is evaluated.
Condition1/Boolean Value1 | Logical AND (&&) | Condition2/Boolean Value2 | Result of Logical AND (&&) |
True | && | True | True |
False | && | True | False |
True | && | False | False |
False | && | False | False |
We can understand that Logical AND returns True only if both operands of it are True and returns False in remaining cases.
//code showing how logical && works with numbers, variables and conditions
void main()
{
int x,y;
x=4&&5; //x will be 1
y=x&&0; //y will be 0
if((x==1)&&(y==0)) //True AND True returns True
printf(“done”); //Hence printed
}
Logical OR
Logical OR (||) checks two boolean values and returns either True or False. The following is the table showing how Logical or is evaluated.
Condition1/Boolean Value1 | Logical OR (||) | Condition2/Boolean Value2 | Result of Logical OR (||) |
True | || | True | True |
False | || | True | True |
True | || | False | True |
False | || | False | False |
We can understand that Logical OR returns False only if both operands of it are False and returns True in remaining cases.
//code showing how logical || works with numbers, variables and conditions
void main()
{
int x,y;
x=4||5; //x will be 1
y=x||0; //y will be 1
if((x==1)||(y==0)) //True OR False returns True
printf(“done”); //Hence printed
}
Logical NOT
Logical NOT (!) is an unary operator that checks boolean value of its operand and returns either True or False. The following is the table showing how Logical NOT is evaluated.
Logical NOT (!) | Condition/Boolean Value | Result of Logical NOT (!) |
! | True | False |
! | False | True |
We can understand that Logical NOT returns False when the operand is True and returns True when the operand is False.
//code showing how logical ! works with numbers, variables and conditions
void main()
{
int x,y;
x=!4; //x will be 0
y=!x; //y will be 1
if(!(y==0)) // NOT False returns True
printf(“done”); //Hence printed
}
Bitwise Operators
These operators are used to perform bit wise operations on the operands. In other words, bitwise operators work on binary representation of the value. Except NOT(~) operator all the remaining bitwise operators are binary operators. Bitwise operators only work with integer data type not with real values. Bitwise operators can work with numbers, variables and even conditions.
Operator Symbol | Operation |
& | AND |
| | OR |
^ | XOR |
~ | NOT/COMPLIMENT |
>> | RIGHT SHIFT |
<< | LEFT SHIFT |
Bitwise AND (&)
Bitwise AND works similar to Logical AND in the evaluation but the difference is Bitwise AND works on the binary representation of operand.
Difference between Logical AND (&&) and Bitwise AND (&)
In the case of numbers or variables, Logical AND(&&) works on the boolean values(non-zero is treated as ‘1’) while Bitwise AND works on binary representation.
4&&7, 4&7
In the case of conditions, Logical AND(&&) works on the short-circuit AND while Bitwise AND works as regular AND evaluation.
a>b && b>c
a>b & b>c
&& is known as short-circuit operator since it may or may not evaluate 2nd argument based on the evaluation of its 1st argument.
Left Shift Operator
This is binary operator that moves each bit in the binary representation of given number towards left to specified number of positions. The blank trailing positions will be filled with ‘0’ after left shift operation.
//example code how left shift operator works
#include
void main()
{
int var=3;
printf(“%d\n”,var);
var=var<<1;
printf(“%d”,var); //output 6
}
Left shifting is equivalent to multiplication of left operand by 2 power right operand.
In the above example, 3<<1 means 3×21
Right Shift Operator
#include
void main()
{
int var=3;
printf(“%d\n”,var);
var=var>>1;
printf(“%d”,var); //OUTPUT 1
}
Right shifting is equivalent to division of left operand by 2 power right operand.
In the above example 3>>1 means 3/21
CONTROL STATEMENTS
Every line in the program has equal priority for its execution. If nothing is specified as constraint all the lines in the program are read and execute top to bottom. This is known as regular or normal flow of program execution. Control statements are used to change the normal flow of program control.
3 types of control statements:
- Selection/decision/branch statements
- Loop/iteration/repetition statements
- Jump statements
DECISION CONSTRUCTS
Selection or Branching means executing different sections of code depending on specific condition.
The following are the different selection constructs of C Language:
Simple if
Multiple if
Nested if
If…else
Switch…case
LOOP STATEMENTS
Loop or Iteration Statements:
Loop control statements are used when a section of code may either be executed a fixed number of times, or while some condition is true.
Three Loop structures are:
- The while loop keeps repeating an action until an associated condition returns false. This is useful where the programmer does not know in advance how many times the loop will be traversed.
- The do while loop is similar, but the condition is checked after the loop body is executed. This ensures that the loop body is run at least once.
- The for loop is used usually where the loop will be traversed a fixed number of times.
Every loop statement must have the following three requirements:
- Declaration and initialisation of control variable
- Condition based on control variable
- Change of value of control variable within the body of the loop so that it can make loop condition at some value.
Infinite Loop: A loop with no termination condition becomes infinite since it keep running.
while Loop:
The syntax is as follows:
while (test condition)
{
body_of_the_loop;
}
/* Program to calculate factorial of given number */
#include
main( )
{
int x;
long int fact = 1;
printf(“Enter any number to find factorial:\n”); /*read the number*/
scanf(“%d”,&x);
while (x > 0)
{
fact = fact * x; /* factorial calculation*/
x=x-1;
}
printf(“Factorial is %ld”,fact);
}
do…while loop
This loop structure executes the block of statements once irrespective of the condition’s evaluation. It checks the condition before 2nd execution.
General syntax of do…while
…………
…………
do
{
…………
…………
} while(cond);
…………
…………
//code prompts user for non-negative denominator and outputs dividend after division
#include
void main()
{
int n,d;
float r;
printf(“Enter Numerator:”);
scanf(“%d”,&n);
do
{
printf(“Enter Denominator:”);
scanf(“%d”,&d);
}while(d==0);
r=(float)n/(float)d;
printf(“Dividend of your division is %.2f”,r);
}
for Loop:
We understood any kind of loop statement must have three requirements they are declaration and initialisation, condition, modification. In the case of while and do…while loops we have to take care about the above specified steps. The ‘for’ loop simplifies the syntax by including these three steps in its header.
General Syntax of ‘for’ loop
for (initialization; condition; modification)
{
………………
………………
}
//code to check whether the given number is prime or not
#include
void main()
{
int n;
printf(“Enter your number:”);
scanf(“%d”,&n);
for(int i=2;i<n;i++)
{
if(n%i==0)
{
printf(“%d is not prime”,n);
break;
}
else
{
printf(“%d is prime”,n);
break;
}
}
}
Nested Loop
A loop statement exists inside another loop statement is known as nested loop. The loop contains another loop in its body is known as outer loop. Here the inner loop completes all its iterations for each iteration of its outer loop. Hence, we can say the inner loop will be executes n2 times for n iterations of outer loop. Any loop can be nested into any other type of loop. For example For loop can have while loop inside its body.
General syntax:
OuterLoop(cond)
{
InnerLoop(cond)
{
………….
…………
}
}
/* Program to print the pattern */
#include
main( )
{
int i,j;
for (i=1;i<=4;i++)
{
for(j=1;j<=i;j++)
printf(“%d\t”,j);
printf(“\n”);
}
}
Jump Statements
These control statements are used to transfer the control of program execution from one place to another based on a specific condition.
The three Jump control statements of C Language are
- break
- continue
- goto
Break Statement
This is used inside any loop or switch…case block to skip the remaining statements and jump out of current block. We have already learnt how to use break statement inside a switch…case block.
/*Program to calculate smallest divisor of a number */
#include
main( )
{
int div,num,i;
printf(“Enter any number:\n”);
scanf(“%d”,&num);
for (i=2;i<=num;++i)
{
if ((num % i) == 0)
{
printf(“Smallest divisor for number %d is %d”,num,i);
break;
}
}
}
Continue Statement
The ‘continue’ statement is used inside the loop allows to skip the following lines in the loop body for the current iteration and passes the control to the beginning of the loop to continue the execution with the next iteration.
/* Program to print first 20 natural numbers skipping the numbers divisible by 5 */
#include
main( )
{
int i;
for (i=1;i<=20;++i)
{
if ((i % 5) == 0)
continue;
printf(“%d ”,i);
}
}
goto Statment
The goto statement transfers the control of execution from the current point to some other portion of the program.
The syntax is as follows:
…….
…….
label:
…………
………….
if(cond)
goto label;
……………
………
In the above syntax, label is an identifier that is used to label the statement to which control will be transferred. The targeted statement must be preceded by the unique label followed by colon.
label : statement;
/* Program to print 10 even numbers */
#include
main()
{
int i=2;
while(1)
{
printf(“%d ”,i);
i=i+2;
if (i>=20)
goto outside;
}
outside : printf(“over”);
}
Loop with the combination of if and goto
We can also make a loop with the combination of if and goto, without using any of the loop statements we have discussed.
//c program to show how loop can be achieved with if and goto
#include
void main()
{
int n,i=1;
printf(“Enter any number:”);
scanf(“%d”,&n);
printf(“\n\nNatural Numbers upto your number:\n”);
start:
printf(“%d\n”,i);
i++;
if(i<=n)
goto start;
printf(“\nEnd of the program”);
}
FUNCTIONS
A function is a block of logically connected executable statements separated from the rest of the program. It can also be understood as a sub program that works for a specific purpose. Sometimes, a set of statements are to be repeatedly appear in the same program or in another case a code written once is needed in another program. In these cases, a separate function can be defined to keep the required code and use the code wherever we want by invoking the function.
Advantages of defining functions
- improves readability of the main program
- reusability in the same or other programs
C Language is also known as procedure-oriented programming language since every C program looks like a collection of functions. A procedure is also known as a process or sub program or function.
Two types of functions we can see in C Program
- Library or built-in or pre-defined functions Eg: printf, scanf etc
- Functions created based on user requirement are known user-defined functions
Function Calling Mechanism
The function which is calling another function is known as caller function and the function which is being called is known as called function. When a function is called, the control transfers to the called function, which will be executed, and then transfers the control back to the caller function (to the statement following the function call).
The three requirements of every function
- definition – specifying what is to be done when the function is invoked
- declaration of the function (optional sometimes) — introduction of the function along with return type and arguments
- invocation of the function –asking to do something
//Example showing the 3 requirements of function
main() //definition of the main function
{
void func(void); //declaration of function
………………….
func(); //invocation of function
…………….
…………….
……………
func();
…………….
…………….
func();
……………
}
void func(void) //definition of function
{
…………….
…………….
……………
}
Definition of function
Function definition is the specification or the program logic that is to be executed when the function has been invoked. Every function must be defined before its invocation. We are including the header files like stdio.h, conio.h into our programs since they consist the definitions of pre-defined functions like printf() and scanf(). Similar to that, we can also define our functions and use them in the same program or include them in other programs in your project.
Function definition consists two parts:
- Header: specifies the attributes of the function
- Body: specifies the executable logic of the function.
Example of function definition:
int add(int x, int y) //header
{//beginning of body
…..
….
}//end of body
The General format of header of the function looks like below
retValType funcName(DataType Var1, DataType Var2,…,DataType VarN)
where
retValType – The data type of the value that is to be returned by the function to its caller
funcName – The identifier or name of the function. Choose an appropriate name to indicate its purpose.
You can see number of items separated with commas inside parenthesis followed by funcName. Those items are known as arguments or parameters those acts as holders for the values supplied by the caller function. In other words, the caller function has to supply values for each every arguments by the time of calling this function.
The ‘void’ data type
The ‘void’ keyword is acting as special data type in C language since it is not used to declare any variables but only used with function definitions. A data type of return value or argument of a function can be any of basic or user-defined data types. Moreover, a function can accept any number of arguments but can return only one value at time. But in case when a function has to be defined so that it is not accepting any arguments or not return any value then ‘void’ keyword is used in the place of missing arguments.
Example:
void funcName(void)
The above header indicating that the function is not going to accept any values or return any.
The return keyword
Default return type of any function is int. It means, if we have omitted void as return type the caller function would expect an integer value from your function.
When a function is returning some value to its caller the return keyword must be used inside its definition. The return statement must be the last statement in the code.
Syntax of return statement:
return;
return (const);
return const;
return (expr);
return expr;
Types of function definitions
Defining a function is depends upon the logic in developer’s mind. We also understood a function can accept zero to many number of arguments and can returns zero to one return value. Based on this we can say, a function can be defined in four ways.
- No arguments and No return value
- No arguments but with return value
- with arguments and no return value
- with arguments and with return value
//Example of function with No arguments and No return value
#include
void add(void) //definition of the function
{
int x,y,sum;
printf(“Enter any two numbers:”);
scanf(“%d%d”,&x,&y);
sum=x+y;
printf(“sum of your numbers is :%d”,sum);
}
void main()
{
add(); //invocation of function
}
//Example of function with No arguments but with return value
#include
int add(void) //definition of the function
{
int x,y;
printf(“Enter any two numbers:”);
scanf(“%d%d”,&x,&y);
return(x+y);
}
void main()
{
int sum;
sum=add(); //invocation of function
printf(“sum of your numbers is :%d”,sum);
}
//Example of function with arguments but no return value
#include
void add(int x,int y) //definition of the function
{
printf(“sum of your numbers is :%d”,x+y);
}
void main()
{
int a,b;
printf(“Enter any two numbers:”);
scanf(“%d%d”,&a,&b);
add(a,b); //invocation of function
}
//Example of function with arguments and with return value
#include
int add(int x,int y) //definition of the function
{
return x+y;
}
void main()
{
int a,b;
printf(“Enter any two numbers:”);
scanf(“%d%d”,&a,&b);
printf(“sum of your numbers is %d”,add(a,b));
}
Recursive function
A function call itself in its body is called recursive function. A recursive function can be used instead of a loop control. Hence, programmer should take care to avoid infinite loop.
/*program to find factorial of a number-without recursion*/
#include
main ()
{
int n, factorial; //declaration of variables
int fact(int); //declaration of fact function
printf (“Enter any number:\n” );
scanf (“%d”, &n);
factorial = fact (n);
printf (“Factorial is %d\n”, factorial);
}
/* Non recursive function of factorial */
int fact (int n) //formal argument
{
int res = 1, i;
for (i = n; i >= 1; i–)
res = res * i;
return (res);
}
/*Program to find factorial using recursion*/
#include
main()
{
int n, factorial;
int fact(int);
printf(“Enter any number: \n” );
scanf(“%d”,&n);
factorial = fact(n); /*Function call */
printf (“Factorial is %d\n”, factorial); }
/* Recursive function of factorial */
int fact(int n)
{
int res;
if(n == 1) /* Terminating condition */
return(1);
else
res = n*fact(n-1); /* Recursive call */
return(res); }
ARRAYS
Array is a data structure storing a group of elements, all of which are of the same data type.
All the elements of an array share the same name, and they are distinguished from one another with the help of an index.
Syntax of array declaration is as follows:
data-type array_name [constant-size];
Data-type refers to the type of elements you want to store
Constant-size is the number of elements
The following are some of declarations for arrays:
int charr [80];
float farr [500];
int iarr [80];
char charray [40];
There are two restrictions for using arrays in C:
- The amount of storage for a declared array has to be specified at compile time before execution. This means that an array has a fixed size.
- The data type of an array applies uniformly to all the elements; for this reason, an array is called a homogeneous data structure.
/* C program that accept marks in 5 subjects of a student */
#include
void main()
{
int m[5];
for(int i=0;i<5;i++)
{
printf(“Enter marks for subject%d”,i+1);
scanf(“%d”,&m[i]);
}
printf(“\n************************”);
printf(“\n*********MENU***********”);
printf(“\n************************”);
for(int i=0;i<5;i++)
{
printf(“\nSubject%d\t%d”,i+1,m[i]);
}
printf(“\n************************”);
}
INITIALIZATION OF ARRAYS
Arrays can be initialized at the time of declaration. The initial values must appear in the order in which they will be assigned to the individual array elements, enclosed within the braces and separated by commas.
Examples:
int digits [10] = {1,2,3,4,5,6,7,8,9,10};
int digits[ ] = {1,2,3,4,5,6,7,8,9,10};
int vector[5] = {12,-2,33,21,13};
float temperature[10] ={ 31.2, 22.3, 41.4, 33.2, 23.3, 32.3, 41.1, 10.8, 11.3, 42.3};
double width[ ] = { 17.33333456, -1.212121213, 222.191345 };
int height[ 10 ] = { 60, 70, 68, 72, 68 };
MULTI-DIMENSIONAL ARRAYS
In principle, there is no limit to the number of subscripts (or dimensions) an array can have. Arrays with more than one dimension are called multi- dimensional arrays.
The first number in brackets is the number of rows, the second number in brackets is the number of columns. So, the upper left corner of any grid would be element [0][0]. The element to its right would be [0][1], and so on.
Initialization of 2-dimensional arrays:
If you have an m x n array, it will have m * n elements and will require m*n*elementsize bytes of storage. To allocate storage for an array you must reserve this amount of memory. The elements of a two-dimensional array are stored row wise.
int table [ 2 ] [ 5 ] = {{ 1,2,3,4,5 },{6,7,8,9.10}};
String Handling
Character Array
We know array is a group of data elements of same data type. Array can be of any basic data type i.e. int, char, double or float. A character array is nothing but an array declared as character data type.
Eg: char name[5];
String
A string in C Language is also character array but has a special termination character null i.e. \0 as its last element.
Difference between String and Character Array
The basic difference between a string and character array is the array is a group of characters but string is a group of character with additional terminal character. C Language can only accommodate the null character automatically only when a character array has additional space.
//Example code showing basic difference between a character array and string
#include
void main()
{
char city1[3]={‘R’,’J’,’Y’}; //character array
char city2[3]=”RJY”; //character array
char city3[]=”RJY”; //string
char city4[4]={‘R’,’J’,’Y’,’\0′}; //string
printf(“size of city1 %d”,sizeof(city1));
printf(“\nsize of city2 %d”,sizeof(city2));
printf(“\nsize of city3 %d”,sizeof(city3));
printf(“\nsize of city4 %d”,sizeof(city4));
}
Advantages of Strings over character array
Basically we use character arrays to store group of characters indicates meaningful words or phrases. After then, we want to perform some operations on the words or phrases like getting the size of word, comparing two words, appending one word to another etc. These operations are complex when you store them as character array. As a solution to this problem, C Language is providing a special header file called ‘string.h’ with many built-in functions to work with strings. These functions may not work correctly with character arrays.
//Example code showing advantage of strings over character arrays
#include
#include
void main()
{
char city1[3]={‘R’,’J’,’Y’}; //character array
char city2[3]=”RJY”; //character array
char city3[]=”RJY”; //string
char city4[4]={‘R’,’J’,’Y’,’\0′}; //string
printf(“\n\n”);
printf(“size of city1 %d”,strlen(city1));
printf(“\nsize of city2 %d”,strlen(city2));
printf(“\nsize of city3 %d”,strlen(city3));
printf(“\nsize of city4 %d”,strlen(city4));
printf(“\n\n”);
strcat(city3,city4);
printf(“\n%s”,city3);
strcat(city1,city2);
printf(“\n%s”,city1);
}
Reading and Writing Strings
Since character array and string are group of characters we have to use the format specifier %s instead of %c to perform read or write operations on the character array or string using standard i/o functions(i.e. printf and scanf). C language also providing some other functions which are only works with strings or character arrays like gets(), puts(), getch(), putch() etc. These functions are also known as unformatted i/o functions.
//Example code showing how to read or write into character array
#include
void main()
{
char name[5];
printf(“Enter your name”);
scanf(“%s”,name); //note there is no subscript or & is used for ‘name’
printf(“\nHi %s, Welcome”,name);
}
When you running the above program, we may observe you could not print the second word in your name. This is because scanf() function only takes input until a delimiter like space has inputted.
Change the scanf() in the above program like below and try again
scanf(“%[^n]s”,name);
You can also unformatted i/o function gets() as a solution to the above problem which will be discussed later in this chapter.
//Example code to get an Enter from User
#include
void main()
{
printf(“press Enter:”);
scanf(“%[^\n]”);
printf(“\nThank you…”);
}
Unformatted I/O
Unformatted I/O in C language is done by the functions like getch, getche, putch, purchar() etc. These functions directly expect a character or text input from the user or print text based output on to the console. No format specifiers will be used for these functions.
getchar()
This function gets the character from user and stores in the variable. It doesn’t accept input until pressed Enter.
#include
main()
{
printf(“Enter a character:”);
char a=getchar();
printf(“\nThe character is %c”,a);
}
puts() and putchar()
It writes the entire sentence, which is in double quotes to the screen. It supports escape sequences but not format specifiers. The putchar() writes its character argument to the screen.
#include
main()
{
char a;
puts(“Enter a character:”);
a=getchar();
puts(“\nThe character entered is”);
putchar(a);
}
getche()
This function assigns the character obtained to the variable, by displaying it on the screen (‘e’ stands for echoing) while getch() returns the obtained character without echoing.
#include
main()
{
char a;
puts(“Enter a character:”);
a=getche(); //replace the getche() with getch() and observe the difference
printf(“\nThe character entered is %c”,a);
}
gets()
The function takes entire text string or phrase from the user and inserts into a character array or string.
//code demonstrates working of gets() function
#include
main()
{
char name[10];
puts(“Enter your Name:”);
gets(name);
printf(“\nHello %s, Welcome to C programming”,name);
}
Strings Handling Functions
As we learnt that C has provided many built-in functions to work with strings, we will continue to know one by one with example programs.
Strlen Function
The strlen function returns the length of a string. It takes the string name as argument.
The syntax is as follows:
n = strlen (str);
where str is name of the string and n is the length of the string, returned by strlen function.
/* Program to illustrate the strlen function to determine the length of a string */
#include
#include
main()
{
char name[80];
int length;
printf(“Enter your name: ”);
gets(name);
length = strlen(name);
printf(“Your name has %d characters\n”, length);
}
Strcpy Function
In C, you cannot simply assign one character array to another. You have to copy element by element. The string library contains a function called strcpy for this purpose. The strcpy function is used to copy one string to another.
The syntax is as follows:
strcpy(str1, str2);
where str1, str2 are two strings. The content of string str2 is copied on to string str1.
/* Program to illustrate strcpy function*/
#include
#include
main()
{
char first[80], second[80];
printf(“Enter a string: ”);
gets(first);
strcpy(second, first);
printf(“\n First string is : %s, and second string is: %s\n”, first, second);
}
Strcmp Function
The strcmp function in the string library function which compares two strings, character by character and stops comparison when there is a difference in the ASCII value or the end of any one string and returns ASCII difference of the characters that is integer. If the return value zero means the two strings are equal, a negative value means that first is less than second, and a positive value means first is greater than second.
The syntax is as follows:
n = strcmp(str1, str2);
where str1 and str2 are two strings to be compared and n is returned value of differed characters.
/* The following program uses the strcmp function to compare two strings. */
#include
#include
main()
{
char first[80], second[80];
int value;
printf(“Enter a string: “);
gets(first);
printf(“Enter another string: “);
gets(second);
value = strcmp(first,second);
if(value==0)
printf(“strings are same”);
else
{
if(value==1)
printf(“second string is big”);
else
{
if(value==-1)
printf(“first string is big”);
}
}
}
strcat Function
The strcat function is used to join one string to another. It takes two strings as arguments; the characters of the second string will be appended to the first string.
The syntax is as follows:
strcat(str1, str2);
where str1 and str2 are two string arguments, string str2 is appended to string str1.
/* Program for string concatenation*/
#include
#include
main()
{
char first[80], second[80];
printf(“Enter a string:”);
gets(first);
printf(“Enter another string: ”);
gets(second);
strcat(first, second);
printf(“\nThe two strings joined together: %s\n”, first);
}
strlwr Function
The strlwr function converts upper case characters of string to lower case characters.
The syntax is as follows:
strlwr(str1);
where str1 is string to be converted into lower case characters.
/* Program that converts input string to lower case characters */
#include
#include
main()
{
char first[80];
printf(“Enter a string: “);
gets(first);
printf(“Lower case of the string is %s”, strlwr(first));
}
strrev Function
The strrev funtion reverses the given string.
The syntax is as follows:
strrev(str);
where string str will be reversed.
/* Program to reverse a given string */
#include
#include
main()
{
char first[80];
printf(“Enter a string:”);
gets(first);
printf(“\n Reverse of the given string is : %s ”, strrev(first));
}
POINTERS
The Pointer is simply a special variable that can hold the address of another variable. In general, every variable holds some kind of data based on its declaration. The declaration of pointer variable also looks like the declaration of regular variable but the difference is pointer stores memory address of a variable but not data.
Declaration of pointer variable
As we know the declaration of pointer variable is almost same as the declaration of regular variable. The general declaration of pointer variable is:
*Identifier;
where:
datatype – any basic or user defined data type
Identifier – any valid identifier following naming conventions
Example:
int *ptr;
You can see the asterisk ‘*’ before the identifier of the pointer variable. The asterisk ‘*’ is not part of the pointer name but indicates that it is a pointer. The data type you can see before the name of the pointer specifying the data type of the variable whose address can be stored by your pointer.
Example:
int x;
float y;
int *ptr;
ptr=&x; //valid
ptr=&y; //invalid
The Address Of and Indirection Operators
The Address Of operator & preceding a variable returns the address of the variable associated with it. Other unary pointer operator is the “*”, also called as value at address or indirection operator. It returns a value stored at that address.
/* Program to print the address associated with a variable and value stored at that address*/
main( )
{
int qty = 5;
printf (“Address of qty = %u\n”,&qty);
printf (“Value of qty = %d \n”,qty);
printf(“Value of qty = %d”,*(&qty));
}
OUTPUT
Address of qty = 65524
Value of qty = 5
Value of qty = 5
Pointer Type Declaration and Assignment
Like other variable, pointer must be declared first before it is used. The significant item to remember here is the data type specified in the declaration of pointer determines the data type of variable whose memory address is stored in the pointer.
Syntax to declare a pointer variable:
datatype *var_name;
Where * informs the compiler to declare a pointer variable
/*program describing pointers*/
# include
main( )
{
int qty = 5;
int *ptr; /* declares ptr as a pointer variable that points to an integer variable*/
ptr = &qty; /* assigning qty’s address to ptr -> Pointer Assignment */
printf (“Address of qty = %u \n”, &qty);
printf (“Address of qty = %u \n”, ptr);
printf (“Address of ptr = %u \n”, &ptr);
printf (“Value of ptr = %d \n”, ptr);
printf (“Value of qty = %d \n”, qty);
printf (“Value of qty = %d \n”, *(&qty));
printf (“Value of qty = %d”, *ptr);
}
OUTPUT
Address of qty = 65524
Address of ptr = 65522
Value of ptr = 65524
Value of qty = 5
Value of qty = 5
Value of qty = 5
NULL Pointer
A pointer that is assigned NULL is called a null pointer.
#include
void main()
{
int a=10;
int *pa;
pa=&a;
printf(“address of a is %d”,pa);
printf(“\nvalue at a is %d”,*pa);
pa=NULL;
printf(“\n\n”);
printf(“address of a is %d”,pa);
printf(“\nvalue of a is %d”,*pa);
}
Pointer Arithmetic
A pointer in c is an address, which is a numeric value. Therefore, you can perform arithmetic operations on a pointer just as you can on a numeric value. There are four arithmetic operators that can be used on pointers: ++, –, +, and –
#include
void main()
{
int a=10;
int *pa;
pa=&a;
printf(“address stored in pa is %d”,pa);
printf(“\nvalue referring by pa is %d”,*pa);
pa++;
printf(“\n\n”);
printf(“address stored in pa is %d”,pa);
printf(“\nvalue referring by pa is %d”,*pa);
pa=pa+2;
printf(“\n\n”);
printf(“address stored in pa is %d”,pa);
printf(“\nvalue referring by pa is %d”,*pa);
}
Relationship of Pointers with Arrays
The name of the array is the implicit pointer to the first variable of array. For example, for an array arr[5],
arr=&arr[0]
arr+1=&arr[1]
….
Arr+4=&arr[4]
//example code comparing address of array with its implicit pointers
#include
int main () {
int var[] = {10, 100, 200};
for ( int i = 0; i < 3; i++)
{
if((var+i)==&var[i])
printf(“\nTRUE”);
}
return 0;
}
//Example code showing how array name can work as pointer
#include
int main () {
int var[] = {10, 100, 200};
for ( int i = 0; i < 3; i++)
{
printf(“Address of var[%d] = %x\n”, i, var+i );
printf(“Value of var[%d] = %d\n”, i, *(var+i) );
}
return 0;
}
Array of Pointers
It is also possible to create an array of pointers and again each pointer is pointing to individual elements of an array. The following example code giving that scenario.
//example code showing how array of pointers can be created
#include
int main () {
int arr[] = {10, 100, 200};
int *pa[3]; //array of pointers
for ( int i = 0; i < 3; i++)
{
pa[i]=&arr[i];
}
for ( int i = 0; i < 3; i++)
{
printf(“\naddress of arr[%d] is : %d”,i,pa[i]);
printf(“\nvalue at arr[%d] is : %d”,i,*(pa[i]));
}
return 0;
}
Pointer to a Pointer
A pointer can hold the address of another pointer variable. Then it will be called as pointer to pointer.
/* Program that declares a pointer to a pointer */
# include
void main( )
{
int i = 100;
int *pi;
int **pii;
pi = &i;
pii = π
printf (“Address of i = %u \n”, &i);
printf (“Address of i = %u \n”, pi);
printf (“Address of i = %u \n”, *pii);
printf (“Address of pi = %u \n”, &pi);
printf (“Address of pi = %u \n”, pii);
printf (“Address of pii = %u \n”, &pii);
printf (“Value of i = %d \n”, i);
printf (“Value of i = %d \n”, *(&i));
printf (“Value of i = %d \n”, *pi);
printf (“Value of i = %d”, **pii);
}
Pointers as Function Arguments
Like regular variables, pointer can also be passed as arguments to the function. We know function accept arguments from their caller function at the time of invocation. When local variables of caller functions are passed as arguments
, a copy of the values of actual arguments is passed to the calling function. Thus, any changes made to the variables inside the function will have no effect on variables used in the actual argument list.
However, when arguments are passed by reference (i.e. when a pointer is passed as an argument to a function), the address of a variable is passed. The contents of that address can be accessed freely, either in the called or calling function. Therefore, the function called by reference can
change the value of the variable used in the call.
/* Program that illustrates the difference between ordinary arguments, which are
passed by value, and pointer arguments, which are passed by reference */
# include
main()
{
int x = 10;
int y = 20;
void swapVal ( int, int ); /* function prototype */
void swapRef ( int *, int * ); /*function prototype*/
printf(“PASS BY VALUE METHOD\n”);
printf (“Before calling function swapVal x=%d y=%d”,x,y);
swapVal (x, y); /* copy of the arguments are passed */
printf (“\nAfter calling function swapVal x=%d y=%d”,x,y);
printf(“\n\nPASS BY REFERENCE METHOD”);
printf (“\nBefore calling function swapRef x=%d y=%d”,x,y);
swapRef (&x,&y); /*address of arguments are passed */
printf(“\nAfter calling function swapRef x=%d y=%d”,x,y);
}
/* Function using the pass by value method*/
void swapVal (int x, int y)
{
int temp;
temp = x;
x = y;
y = temp;
printf (“\nWithin function swapVal x=%d y=%d”,x,y);
return;
}
/*Function using the pass by reference method*/
void swapRef (int *px, int *py)
{
int temp;
temp = *px;
*px = *py;
*py = temp;
printf (“\nWithin function swapRef *px=%d *py=%d”,*px,*py);
return;
}
Pointer as Function Return value
A pointer can also be returned by a function as its return value to its caller. Using this method a function can able change more than one values in the caller function, which is usually not possible in regular method.
/*Program that shows how a function returns a pointer */
# include
void main( )
{
float *a;
float *func( ); /* function prototype */
a = func( );
printf (“Address = %u”, a);
}
float *func( )
{
float r = 5.2;
return (&r);
}
STRUCTURES AND UNIONS
We learnt about basic data types of C language i.e. int, float, double and char. Those are useful to specify the characteristics of variable declared out of them. For example, the data type ‘int’ specifies 2 bytes of memory to be allocated and rounded numbers only allowed to store in that memory. But is there any way to define our own data type in C language?
We understood that we can declare an array out of any basic data types. But all the elements of array can store only same type of data. What is the solution when we want to store different types of related data element as a single item. For example, we want to store a record of student i.e. his name, RollNo, marks etc. You can understand name can be declared as character string, rollno as integer and marks as float. But these three variables belong to same person. It is worth store them a single variable. But this cannot be stored as an array. The solution of above mentioned two problems is defining our own data type.
What is a Structure?
Structure in C language allows us to define user-defined data types and then declare variables out of them. Each structure can have different data items of different types. Each variable declared out of the structure have a copy of all the data items in structure definition. Each data item existed in structure definition are known as members of structure. The variable declared out of the structure can access its copy of members with dot (.) operator.
Data type vs data structure
Data type is a keyword used to specify characteristics and valid operations for the variable declared out it. But a data structure is an arrangement of data which can be any basic or user-defined data type. Data structure specifies relationship between data elements regarding their storage and accessibility. Array is homogenous data structure while Structure is heterogenous user-defined data type.
There are three syntaxes to define a structure data type.
Syntax1:
struct structName
{
Datatype Member1;
Datatype Member2;
…
Datatype member N;
};
struct structName var1; //declaration of structure variable
Syntax2:
struct structName
{
Datatype Member1;
Datatype Member2;
…
Datatype member N;
}var1;
Syntax3:
typedef struct
{
Datatype Member1;
Datatype Member2;
…
Datatype member N;
}structName;
structName var1;
//Example program showing how structure can be defined and used
#include
typedef struct
{
int sno;
float m1,m2,m3;
}student;
/*program*/
void main()
{
student st={123,80,90,70};//declaration and initialisation of student variable st
void display(int, float, float, float); //declaration of function display()
display(st.sno,st.m1,st.m2,st.m3); //invocation of display function
}
void display(int sno,float s1, float s2, float s3) //definition of display function
{
printf(“\nrollNo: %d\nsub1 : %f\nsub2 : %f\nsub3 : %f”,sno,s1,s2,s3);
}
/*Program to store and retrieve the values from the student structure*/
#include
typedef struct{ //definition of student structure(datatype)
int roll_no;
char name[20];
char course[20];
float marks_obtained ;
}student;
void main()
{
student s1 ; //declaration of student variable s1
printf (“Enter the student roll number:”);
scanf (“%d”,&s1.roll_no);
printf (“\nEnter the student name: “);
scanf (“%s”,s1.name);
printf (“\nEnter the student course”);
scanf (“%s”,s1.course);
printf (“Enter the student percentage\n”);
scanf (“%f”,&s1.marks_obtained);
printf (“\nData entry is completed”);
printf ( “\nThe data entered is as follows:\n”);
printf (“\nThe student roll no is %d”,s1.roll_no);
printf (“\nThe student name is %s”,s1.name);
printf (“\nThe student course is %s”,s1.course);
printf (“\nThe student percentage is %f”,s1.marks_obtained);
}
INITIALIZING STRUCTURES
/* Program to illustrate to access the values of the structure initialized with some
initial values*/
#include
struct telephone{
int tele_no;
int cust_code;
char cust_name[20];
char cust_address[40];
int bill_amt;
};
main()
{
struct telephone tele = {2314345,
5463,
“Ram”,
“New Delhi”,2435 };
printf(“The values are initialized in this program.”);
printf(“\nThe telephone number is %d”,tele.tele_no);
printf(“\nThe customer code is %d”,tele.cust_code);
printf(“\nThe customer name is %s”,tele.cust_name);
printf(“\nThe customer address is %s”,tele.cust_address);
printf(“\nThe bill amount is %d”,tele.bill_amt);
}
STRUCTURE ARRAYS
Array declared out any basic data type can be a member in a structure and even array of structure can also be declared.
/*Program to read and print the data for 3 students*/
#include
struct student
{
int roll_no;
char name[20]; //array member
char course[20];
int marks_obtained ;
};
void main( )
{
struct student stud[3];
int i;
printf (“Enter the student data one by one\n\n”);
for(i=0; i<3; i++)
{
printf (“Enter the roll number of student%d :”,i+1);
scanf (“%d”,&stud[i].roll_no);
printf (“Enter the name of student%d :”,i+1);
scanf (“%s”,stud[i].name);
printf (“Enter the course of student%d :”,i+1);
scanf (“%s”,stud[i].course);
printf (“Enter the marks obtained of student%d :”,i+1);
scanf (“%d”,&stud[i].marks_obtained);
printf(“\n”);
}
printf (“the data entered is as follows\n”);
for (i=0;i<3;i++)
{
printf (“The roll number of student%d is %d :\n”,i+1,stud[i].roll_no);
printf (“The name of student%d is %s :\n”,i+1,stud[i].name);
printf (“The course of student%d is %s :\n”,i+1,stud[i].course);
printf (“The marks of student%d is %d :\n”,i+1,stud[i].marks_obtained);
}
}
STRUCTURES AS FUNCTION ARGUMENTS
Since structure variables are also like regular variables declared out of a certain data types, they can be passed to functions as arguments.
/*Example Program to demonstrate passing a structure to a function*/
#include
/*Declare and define a structure to hold the data.*/
struct data{
float amt;
char fname [30];
char lname [30];
}per; //declaration of per which is the global variable of data
void main()
{
void printPer (struct data); //declaration of print_per function
printf(“Enter the donors first and last names separated by a space:”);
scanf (“%s %s”, per.fname, per.lname);
printf (“\nEnter the amount donated in rupees:”);
scanf (“%f”, &per.amt);
printPer (per); //invocation of function print_per
}
void printPer(struct data x) //definition of function print_per
{
printf (“\n %s %s gave donation of amount Rs.%.2f\n”, x.fname, x.lname, x.amt);
}
STRUCTURE AS FUNCTION RETURN VALUE
/* Example Program to show how structure variable can be returned by a function
The purpose of the program is to accept the data from the user and calculate the salary of the person*/
#include
struct sal { //definition of sal structure
char name[30];
int no_days_worked;
int daily_wage;
};
int main()
{
struct sal salary; //salary is the variable of sal type
float amount_payable; /* variable declaration*/
struct sal get_data(void); /* function prototype*/ //declaration of function get_dat
float wages(struct sal); /*function prototype*/
salary = get_data(); //invoking get_data function by passing salary variable
printf(“\n\nThe name of employee is %s”,salary.name);
printf(“\nNumber of days worked is %d”,salary.no_days_worked);
printf(“\nThe daily wage of the employees is %d”,salary.daily_wage);
amount_payable = wages(salary);
printf(“\n\nThe amount payable to %s is %.2f”,salary.name,amount_payable);
return 0;
}
struct sal get_data() //definition of get_data function
{
struct sal income; //income is a variable of sal type
printf(“Please enter the employee name:\n”);
scanf(“%s”,income.name);
printf(“Please enter the number of days worked:\n”);
scanf(“%d”,&income.no_days_worked);
printf(“Please enter the employee daily wages:\n”);
scanf(“%d”,&income.daily_wage);
return(income);
}
float wages(struct sal amt)
{
float total_salary ;
total_salary = amt.no_days_worked * amt.daily_wage;
return(total_salary);
}
STRUCTURE POINTER
A pointer of basic or user-defined data type can be member of a structure and even a pointer to a structure can be created.
//Example program to show how a pointer can be a member of a structure
#include
struct data
{
int x; //Stores address of integer Variable
int *ptr;
};
int main()
{
struct data d1;
d1.ptr=&d1.x;
*d1.ptr=10;
printf(“Value of x inside d1 is %d”,*d1.ptr);
return(0);
}
When a pointer to structure type is created it can store address of any variable of the same structure. Now the question is how to access the members of structure using pointer. For this, there are two solutions.
- Using * and . operators together
- Using arrow(->) or membership operator
//Example program to show how a pointer to a structure can be created
#include
struct data
{
int x,y; //Stores address of integer Variable
};
int main()
{
struct data *sptr,d1;
sptr=&d1;
(*sptr).x=10;
sptr->y=20;
printf(“Value of x inside d1 is %d”,sptr->x);
printf(“\nValue of y inside d1 is %d”,(*sptr).y);
return(0);
}
STRUCTURE AS MEMBER OF ANOTHER STRUCTURE
A well-defined structure variable can be a member of another structure and even a structure definition can exists inside another structure (inner structure).
UNIONS
Union is another way of creating user-defined data type in C language. We can create union data type just by using ‘union’ keyword instead of ‘struct’. Union data type also contains number of data members of basic or user-defined data types. Each union variable declared out of union data type can access its member using dot (.) operator. Then what are the differences between structures and unions? We will look at those interesting points below.
Differences between structure and union
- Structure can be defined with ‘struct’ keyword while union can be defined with ‘union’ keyword
- The memory size of the variable of structure data type is greater than or equal to the total size of its members size. The memory size of the variable of union data type is equal to the memory requirement of one data member who need highest memory.
- Each data member of structure has individual memory allocation within its variable’s memory. All data members of union share the same memory allocated for union variable.
- Altering value of one data member of structure won’t effect another data member. Altering value of one data member of union will effect another data members too.
- The data members of structure can be accessed at a time but only one member of union can be accessed
- Initialisation of more than one member of structure is possible but only the first member of union is possible.
//Example program to show differences between structure and union
#include
typedef struct
{
char name[10];
int age;
float marks;
} stdstruct;
typedef union
{
char name[10];
int age;
float marks;
}stdunion;
int main()
{
stdstruct s1;
stdunion s2;
printf(“Enter values for structure variable:”);
printf(“\nEnter name of the student:”);
scanf(“%s”,s1.name);
printf(“Enter age:”);
scanf(“%d”,&s1.age);
printf(“Enter marks:”);
scanf(“%f”,&s1.marks);
printf(“\n\n”);
printf(“Enter values for union variable:”);
printf(“\nEnter name of the student:”);
scanf(“%s”,s2.name);
printf(“Enter age:”);
scanf(“%d”,&s2.age);
printf(“Enter marks:”);
scanf(“%f”,&s2.marks);
printf(“\n\n”);
printf(“Values for structure variable:”);
printf(“\nName of the student: %s”,s1.name);
printf(“\nAge: %d”,s1.age);
printf(“\nMarks: %f”,s1.marks);
printf(“\n\n”);
printf(“Values for union variable:”);
printf(“\nName of the student: %s”,s2.name);
printf(“\nAge: %d”,s2.age);
printf(“\nMarks: %f”,s2.marks);
printf(“\n\n”);
getch();
s1.age=26;
s2.age=21;
printf(“After modifications, Values for structure variable:”);
printf(“\nName of the student: %s”,s1.name);
printf(“\nAge: %d”,s1.age);
printf(“\nMarks: %f”,s1.marks);
printf(“\n\n”);
printf(“After modifications, Values for union variable:”);
printf(“\nName of the student: %s”,s2.name);
printf(“\nAge: %d”,s2.age);
printf(“\nMarks: %f”,s2.marks);
return(0);
}
FILE HANDLING
When data is stored using variables, the data is lost when the program exits unless something is done to save it. C views file simply as a sequential stream of bytes. Each file ends either with an end-of-file marker or at a specified byte number recorded in a system maintained, administrative data structure. C supports two types of files called binary files and text files.
The difference between these two files is in terms of storage. In text files, everything is stored in terms of text i.e. even if we store an integer 54; it will be stored as a 3-byte string – “54\0”.
A binary file contains data that was written in the same format used to store internally in main memory. For example, the integer value 1245 will be stored in 2 bytes depending on the machine while it will require 5 bytes in a text file.
When the file is opened the stream is associated with the file. Three streams are opened bydefault with program execution:
- standard input: enables program to read data from keyboard
- standard output: enables program to write data on screen
- standard error: enables program to track input and output errors
The above streams are manipulated with the help of pointers called stdin, stdout and stderr.
Further, to access any file, we need to declare a pointer to FILE structure (defined in stdio.h) and then associate it with a particular file. This pointer is referred to as file pointer and is declared as:
FILE *fp;
Opening a file using function fopen()
Once the file pointer has been declared, the next step is to open a file. The fopen() function opens a stream and links a file with the stream. This function returns a file pointer.
Syntax:
FILE *fopen(char *filename,mode);
Different modes for opening a file:
MODE MEANING
r/rt opens a text file for read only access
w/wt opens a text file for write only access
r+t opens a text file for read and write access
w+t creates a text file for read and write access
/*program that tries to open a file*/
#include
void main()
{
FILE *fp;
fp=fopen(“file1.txt”,”r”);
if(fp==NULL)
printf(“FILE DOES NOT EXISTS”);
}
Closing a file using fclose()
The file that has been opened should be closed before exiting the program.
syntax:
int fclose(FILE *fptr);
the return value is 0 if the file successfully closed or EOF if an error occurred. If the fclose() function is not called the operating system normally close the file when the program execution terminates.
//creating a file with fprintf
#include
void main(){
FILE *fp;
fp = fopen(“c:\\users\\satyamitguru\\desktop\\file2.txt”, “w”);//creating a file at a specific location
fprintf(fp, “Hello file by fprintf…\n”);//writing data into file
fclose(fp);//closing file
printf(“Done…”);
}
//Write to a text file
#include
#include
int main()
{
int num;
FILE *fptr;
fptr = fopen(“program.txt”,”w”);
if(fptr == NULL)
{
printf(“Error!”);
exit(1);
}
printf(“Enter num: “);
scanf(“%d”,&num);
fprintf(fptr,”%d”,num);
fclose(fptr);
return 0;
}
//Read from a text file
#include
#include
int main()
{
int num;
FILE *fptr;
if ((fptr = fopen(“program.txt”,”r”)) == NULL){
printf(“Error! opening file”);
// Program exits if the file pointer returns NULL.
exit(1);
}
fscanf(fptr,”%d”, &num);
printf(“Value of n=%d”, num);
fclose(fptr);
return 0;
}